Part 1: Introduction to SuiteCRM API Version 4_1
SuiteCRM provides several API versions to interact with its CRM functionalities. The Version 4_1 API, while older, remains widely used due to its simplicity and broad compatibility with existing systems. This article focuses on Version 4_1 to help you quickly get started with SuiteCRM integrations using Postman.
Version 4_1 is particularly useful for developers who want straightforward solutions without dealing with the complexity of newer protocols. In future articles, we’ll explore more advanced SuiteCRM APIs, including the V8 API and GraphQL, to provide a comprehensive understanding of SuiteCRM’s evolving API landscape.
The SuiteCRM Version 4_1 API is based on a REST-like architecture. Despite being an older API version, it’s still widely used and supports key CRM functionalities. We’ll demonstrate how to:
- Authenticate and obtain a session ID.
- Create a new lead.
- Retrieve a lead by specific criteria.
- Update a lead.
- Create a note and attach a file.
1. Authenticating with SuiteCRM API 4_1
Before we get started, I’d recommend you create a “user” in SuiteCRM specifically for this purpose. Something like “apiuser”. The benefit of this is that you don’t have to use your admin login/password for the API and also in the future you can customize a security group for your API user and give the user access to only modules and functionality you want it to have.
Before making any requests, you need to authenticate and obtain a session ID.
Authentication with form-data in Postman
Of special note, you must use form-data to submit your request. RAW will not work (at least I’ve never been able to make it work).
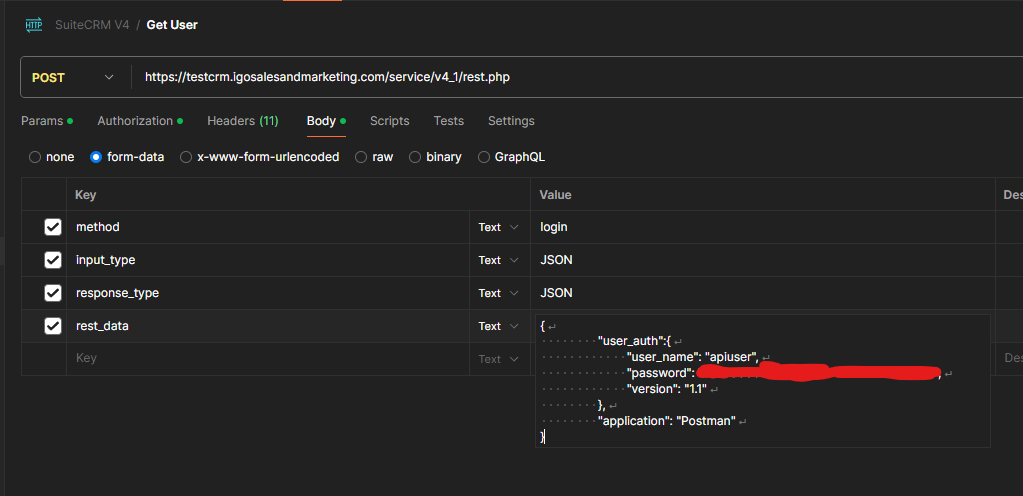
- Method:
POST
- URL:
https://your-suitecrm-instance/service/v4_1/rest.php
- Headers:
Content-Type: application/x-www-form-urlencoded
input_type
:JSON
response_type
:JSON
rest_data
:
{
"user_auth": {
"user_name": "your-username",
"password": "your-md5-hashed-password",
"version": "1.1"
},
"application": "Postman"
}
Response Example:
{
"id": "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
"module_name": "Users",
"name_value_list": {}
}
The id
field in the response is your session ID, which will be required for subsequent requests.
2. Creating a New Lead
create a new lead, you’ll use the set_entry
method.
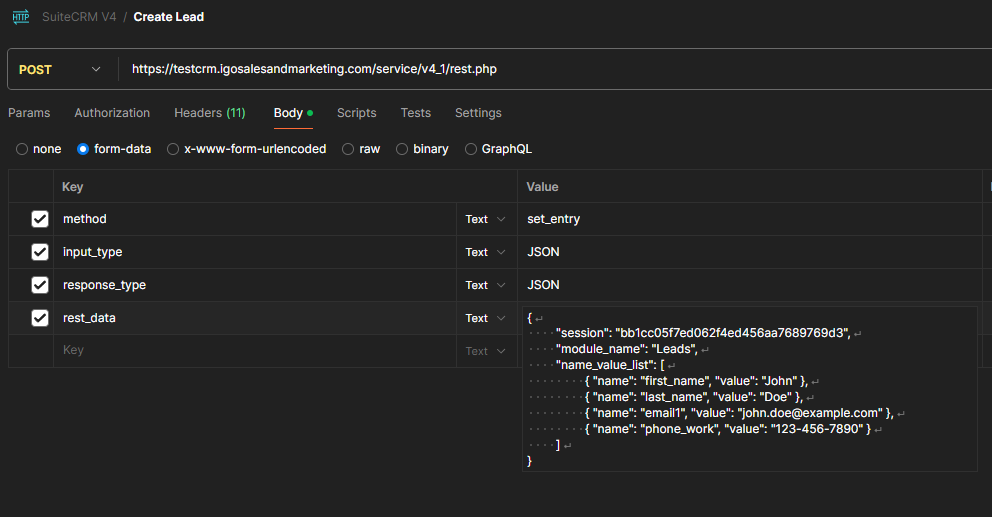
Postman Configuration:
- Method:
POST
- URL:
https://your-suitecrm-instance/service/v4_1/rest.php
- Headers:
Content-Type: application/x-www-form-urlencoded
Body (form-data):
method
:set_entry
input_type
:JSON
response_type
:JSON
rest_data
:
{
"session": "your-session-id",
"module_name": "Leads",
"name_value_list": [
{ "name": "first_name", "value": "John" },
{ "name": "last_name", "value": "Doe" },
{ "name": "email1", "value": "john.doe@example.com" },
{ "name": "phone_work", "value": "123-456-7890" }
]
}
Response Example:
{
"id": "22248b09-fb5b-8d59-0f26-6780374c4e3b",
"entry_list": {
"first_name": {
"name": "first_name",
"value": "John"
},
"last_name": {
"name": "last_name",
"value": "Doe"
},
"email1": {
"name": "email1",
"value": "john.doe@example.com"
},
"phone_work": {
"name": "phone_work",
"value": "123-456-7890"
}
}
}
This response contains the id
of the newly created lead.
3. Retrieving a Lead by First and Last Name
To retrieve a lead by specific criteria (e.g., first name and last name), you can use the get_entry_list
method.
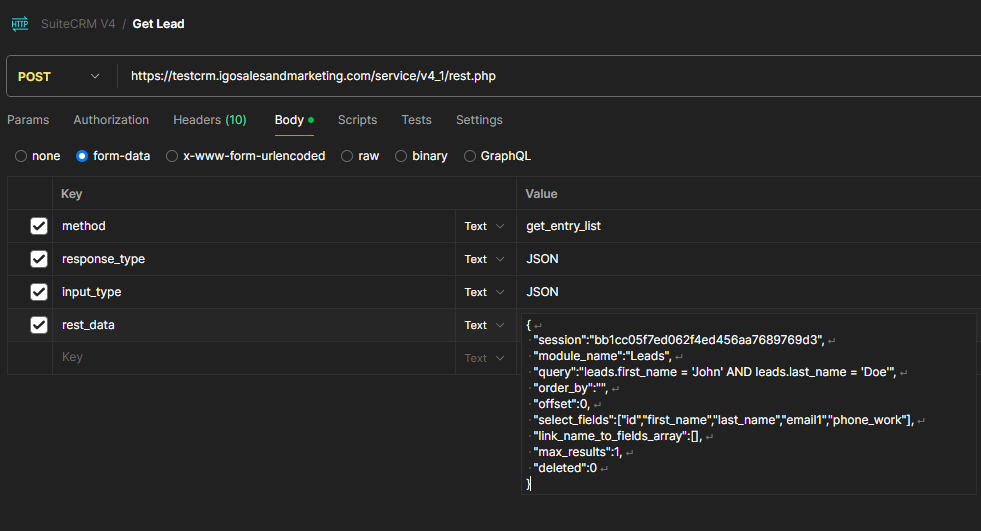
Postman Configuration:
- Method:
POST
- URL:
https://your-suitecrm-instance/service/v4_1/rest.php
- Headers:
Content-Type
:application/x-www-form-urlencoded
Body (form-data):
method
:set_entry
input_type
:JSON
response_type
:JSON
rest_data
:
{
"session":"your-session-id",
"module_name":"Leads",
"query":"leads.first_name = 'John' AND leads.last_name = 'Doe'",
"order_by":"",
"offset":0,
"select_fields":["id","first_name","last_name","email1","phone_work"],
"link_name_to_fields_array":[],
"max_results":1,
"deleted":0
}
Response Example:
{
"result_count": 1,
"total_count": "1",
"next_offset": 1,
"entry_list": [
{
"id": "22248b09-fb5b-8d59-0f26-6780374c4e3b",
"module_name": "Leads",
"name_value_list": {
"id": {
"name": "id",
"value": "22248b09-fb5b-8d59-0f26-6780374c4e3b"
},
"first_name": {
"name": "first_name",
"value": "John"
},
"last_name": {
"name": "last_name",
"value": "Doe"
},
"email1": {
"name": "email1",
"value": "john.doe@example.com"
},
"phone_work": {
"name": "phone_work",
"value": "123-456-7890"
}
}
}
],
"relationship_list": []
}
You can now use the id
from the response to update this lead.
You can now use the id
from the response to update this lead.
4. Updating a Lead
To update the lead, use the set_entry
method again, but include the lead’s ID.
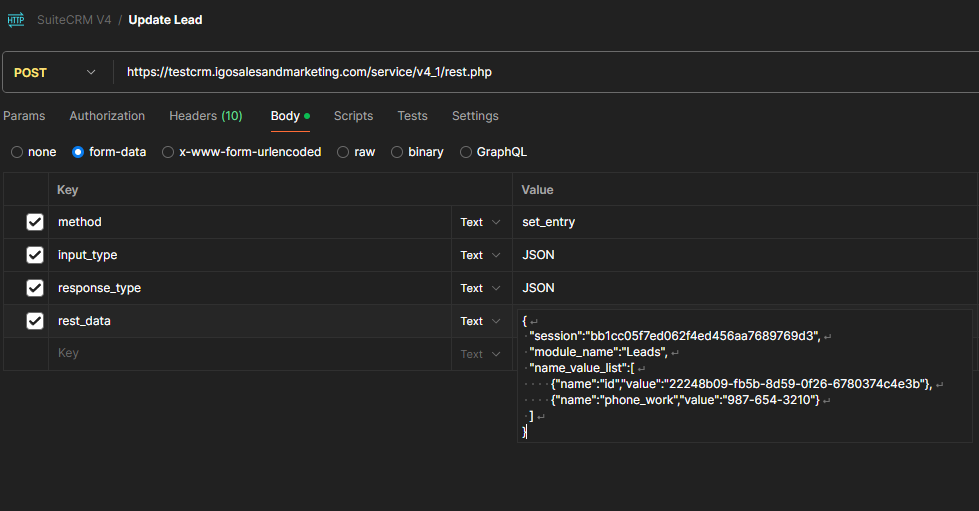
Postman Configuration:
- Method:
POST
- URL:
https://your-suitecrm-instance/service/v4_1/rest.php
- Headers:
Content-Type
:application/x-www-form-urlencoded
Body (form-data):
method
:set_entry
input_type
:JSON
response_type
:JSON
rest_data
:
{
"session":"your-session-id",
"module_name":"Leads",
"name_value_list":[
{"name":"id","value":"lead-id"},
{"name":"phone_work","value":"987-654-3210"}
]
}
Response Example:
{
"id": "22248b09-fb5b-8d59-0f26-6780374c4e3b",
"entry_list": {
"id": {
"name": "id",
"value": "22248b09-fb5b-8d59-0f26-6780374c4e3b"
},
"phone_work": {
"name": "phone_work",
"value": "987-654-3210"
}
}
}
5. Creating a Note and Attaching a File
To attach a file, you first need to create a note linked to the lead. Once the note is created, you can use the set_note_attachment
method to upload a file to the note. We’re going to also relate the Note to the Lead we just created by using parent_id.
Step 1: Create a Note
Postman Configuration:
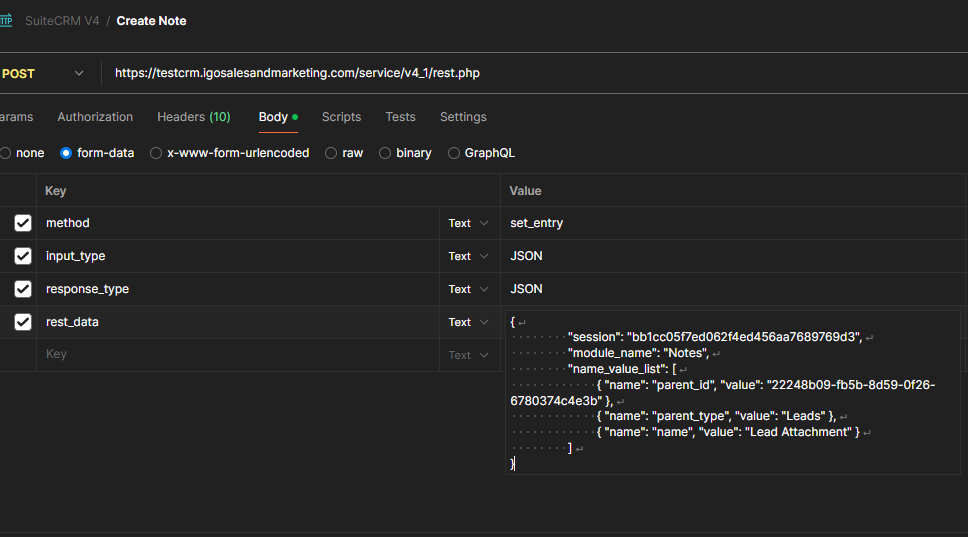
- Method:
POST
- URL:
https://your-suitecrm-instance/service/v4_1/rest.php
- Headers:
Content-Type
:application/x-www-form-urlencoded
Body (form-data):
method
:set_entry
input_type
:JSON
response_type
:JSON
rest_data
:
{
"session": "your-session-id",
"module_name": "Notes",
"name_value_list": [
{ "name": "parent_id", "value": "lead-id" },
{ "name": "parent_type", "value": "Leads" },
{ "name": "name", "value": "Lead Attachment" }
]
}
Reponse Example:
{
"id": "e1b8da4e-f956-014a-2015-678043429291",
"entry_list": {
"parent_id": {
"name": "parent_id",
"value": "22248b09-fb5b-8d59-0f26-6780374c4e3b"
},
"parent_type": {
"name": "parent_type",
"value": "Leads"
},
"name": {
"name": "name",
"value": "Lead Attachment"
}
}
}
Step 2: Attach a File to the Note
Once the note is created, you can attach a file using the set_note_attachment
method.
Postman Configuration:
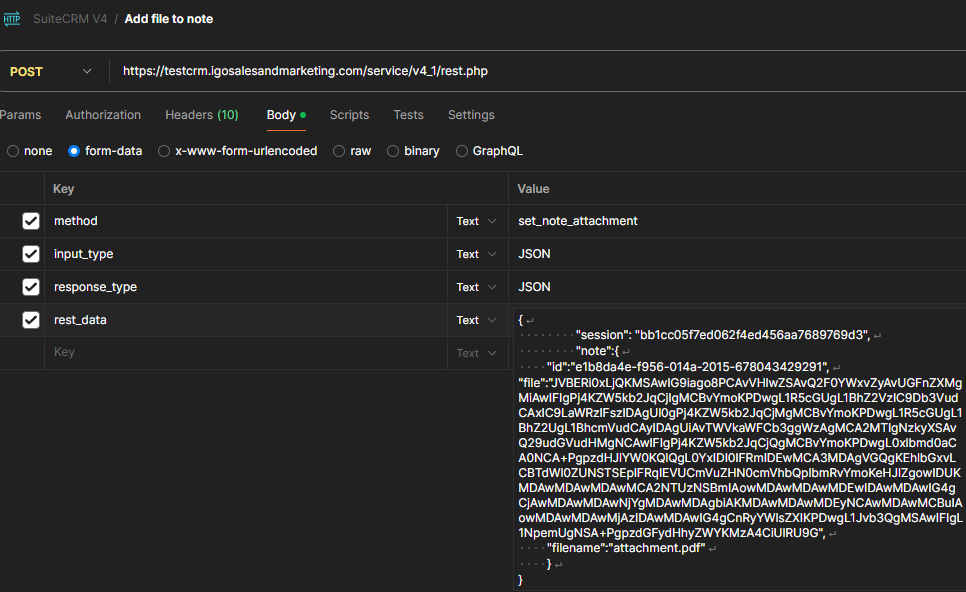
- Method:
POST
- URL:
https://your-suitecrm-instance/service/v4_1/rest.php
- Headers:
Content-Type
:application/x-www-form-urlencoded
Body (raw, JSON):
method
:set_note_attachment
input_type
:JSON
response_type
:JSON
rest_data
:
{
"session":"your-session-id",
"note":{
"id":"note-id",
"file":"base64-encoded-file-content",
"filename":"attachment.pdf"
}
}
To encode a file in Base64, you can use an online tool or your local system. Ensure the encoded content is properly copied. By code, it’s easy just add it to your php function to encode it.
Response Example:
{
"id": "note-id"
}
Documentation
There are plenty of other methods available for the 4_1 API. It would be too long a tutorial to go through every one. Now that you know the basics of how to use Postman you can construct some API calls of your own using some other methods. You can find them here:
https://docs.suitecrm.com/developer/api/api-v4.1-methods
Method | Description |
---|---|
login | Authenticates a user and creates a session ID for subsequent requests. |
logout | Ends a session associated with the provided session ID. |
get_user_id | Retrieves the user ID for the authenticated session. |
get_entry | Fetches a single record from a specified module by ID. |
get_entries | Fetches multiple records from a specified module by their IDs. |
get_entry_list | Retrieves a list of records from a specified module, with optional filtering and pagination. |
set_entry | Creates or updates a single record in a specified module. |
set_entries | Creates or updates multiple records in a specified module. |
get_relationships | Retrieves related records for a specific module record. |
set_relationship | Creates or updates a relationship between records in different modules. |
set_relationships | Creates or updates multiple relationships in different modules. |
get_available_modules | Returns a list of all available modules for the authenticated user. |
get_module_fields | Retrieves metadata about the fields in a specified module. |
get_document_revision | Retrieves a specific document revision by ID. |
set_note_attachment | Attaches a file to an existing note. |
get_note_attachment | Retrieves the attachment file content of a note. |
set_document_revision | Creates a new document revision and uploads a file. |
search_by_module | Searches for records across specified modules using a query string. |
get_report_entries | Retrieves the entries generated by a specific report in SuiteCRM. |